Build 1D model
[1]:
import sys
sys.path.append("../")
import numpy as np
import warmth
from warmth.forward_modelling import Forward_model
[2]:
model = warmth.Model()
[3]:
import pandas as pd
node_template=model.builder.single_node_sediments_inputs_template
h1 = [152.0,0.0,1.500000,2.301755e-09,0.620000,0.500,2720.0,2448.0]
h2=[810.0,20.0,1.538462,2.079433e-09,0.599730,0.490,2708.0,2437.2]
h3=[1608.0,66,1.500000,2.301755e-09,0.2,0.500,2720.0,2448.0]
h4=[1973.0,100,1.500000,2.301755e-09,0.620000,0.500,2720.0,2448.0]
h5=[2262.0,145,1.500000,2.301755e-09,0.620000,0.500,2720.0,2448.0]
h6=[2362.0,152,1.904762,4.719506e-10,0.447705,0.415,2618.0,2356.2]
h7=[2427.0,160,1.500000,2.301755e-09,0.620000,0.500,2720.0,2448.0]
horizons=[h1,h2,h3,h4,h5,h6,h7]
for i in horizons:
new =pd.DataFrame.from_dict({'top': [i[0]], 'topage': [int(i[1])], 'k_cond': [i[2]], 'rhp':[i[3]], 'phi':[i[4]], 'decay':[i[5]], 'solidus':[i[6]],'liquidus':[i[7]]})
node_template = pd.concat([node_template, new], ignore_index=True)
Create a single node and add it to the model
[4]:
node =warmth.single_node()
node.sediments_inputs = node_template
node.sediments
/workspaces/warmth/warmth/build.py:199: FutureWarning: In a future version, the Index constructor will not infer numeric dtypes when passed object-dtype sequences (matching Series behavior)
check_ascending = df.apply(lambda x: x.is_monotonic_increasing)
[4]:
top | topage | k_cond | rhp | phi | decay | solidus | liquidus | base | baseage | thickness | grain_thickness | phi_mean | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 152.0 | 0 | 1.500000 | 2.301755e-09 | 0.620000 | 0.500 | 2720.0 | 2448.0 | 810.0 | 20 | 658.0 | 0.310357 | 0.528332 |
1 | 810.0 | 20 | 1.538462 | 2.079433e-09 | 0.599730 | 0.490 | 2708.0 | 2437.2 | 1608.0 | 66 | 798.0 | 0.511062 | 0.359571 |
2 | 1608.0 | 66 | 1.500000 | 2.301755e-09 | 0.200000 | 0.500 | 2720.0 | 2448.0 | 1973.0 | 100 | 365.0 | 0.332780 | 0.088275 |
3 | 1973.0 | 100 | 1.500000 | 2.301755e-09 | 0.620000 | 0.500 | 2720.0 | 2448.0 | 2262.0 | 145 | 289.0 | 0.221878 | 0.232256 |
4 | 2262.0 | 145 | 1.500000 | 2.301755e-09 | 0.620000 | 0.500 | 2720.0 | 2448.0 | 2362.0 | 152 | 100.0 | 0.078943 | 0.210571 |
5 | 2362.0 | 152 | 1.904762 | 4.719506e-10 | 0.447705 | 0.415 | 2618.0 | 2356.2 | 2427.0 | 160 | 65.0 | 0.053525 | 0.176536 |
[5]:
model.parameters.time_start=int(node.sediments["baseage"].iloc[-1])
model.parameters.time_end = 0
[6]:
node.crustRHP = 2e-6
node.qbase = 30e-3
node.rift = np.array([[160,145]])
#node.paleoWD=np.array([200]) # Only for mulit rift
[7]:
model.builder.nodes = [[node]]
[8]:
from warmth.data import haq87
model.builder.set_eustatic_sea_level(haq87)
[9]:
%%time
model.simulator.run(parallel=False)
CPU times: user 612 ms, sys: 0 ns, total: 612 ms
Wall time: 624 ms
[10]:
model.builder.nodes[0][0].beta
[10]:
array([1.24])
[11]:
%matplotlib inline
import matplotlib.pyplot as plt
plt.plot(node.result.ages,[node.result.basement_heatflow(age)*1000 for age in node.result.ages])
plt.gca().invert_xaxis()
plt.xlabel("Time (Ma)")
plt.ylabel("Basement heat flow (mW/m2)")
plt.show()
/workspaces/warmth/warmth/postprocessing.py:199: RuntimeWarning: invalid value encountered in divide
v=-1*initial_poro/initial_decay*phi1
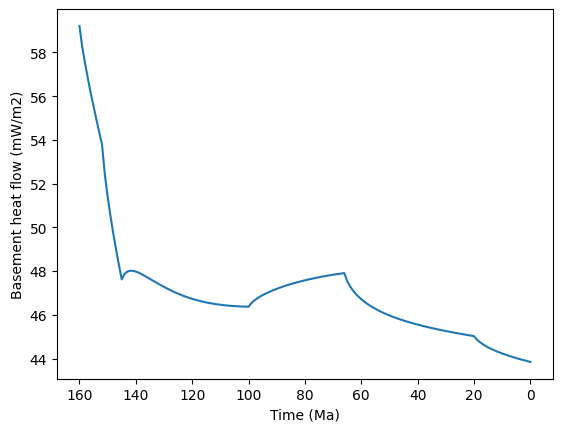
[12]:
import ipywidgets as widgets
import matplotlib.pyplot as plt
def plot_cell_bound(age):
t = node.result.temperature(age)
plt.plot(t["values"],t["depth"])
plt.gca().invert_yaxis()
plt.xlabel("Temperature (C)")
plt.ylabel("Depth (m)")
plt.ylim(top=0,bottom=node.result.temperature(age,-2)["depth"][0]+1000)
plt.show()
widgets.interact(plot_cell_bound, age = widgets.IntSlider(value=0,max=node.result.ages[-1],min=node.result.ages[0],step=1))
[12]:
<function __main__.plot_cell_bound(age)>
[17]:
def plot_cell_properties(age):
t = node.result.effective_conductivity(age)
plt.plot(t["values"],t["depth"])
plt.gca().invert_yaxis()
plt.xlabel("Conductivity")
plt.ylabel("Depth (m)")
plt.ylim(top=0,bottom=node.result.temperature(age,-1)["depth"][0])
plt.show()
widgets.interact(plot_cell_properties, age = widgets.IntSlider(value=0,max=node.result.ages[-1],min=node.result.ages[0],step=1))
[17]:
<function __main__.plot_cell_properties(age)>
[ ]: